Validating email is a very important point while validating an HTML form. In this page we have discussed how to validate an email using JavaScript :
An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. a "personal_info" and a domain, that is personal_info@domain. The length of the personal_info part may be up to 64 characters long and domain name may be up to 253 characters.
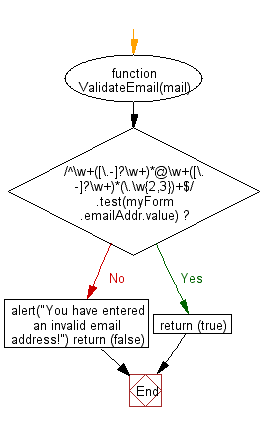
An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. a "personal_info" and a domain, that is personal_info@domain. The length of the personal_info part may be up to 64 characters long and domain name may be up to 253 characters.
The personal_info part contains the following ASCII characters.
- Uppercase (A-Z) and lowercase (a-z) English letters.
- Digits (0-9).
- Characters ! # $ % & ' * + - / = ? ^ _ ` { | } ~
- Character . ( period, dot or fullstop) provided that it is not the first or last character and it will not come one after the other.
The domain name [for example com, org, net, in, us, info] part contains letters, digits, hyphens, and dots.
Example of valid email id
- mysite@ourearth.com
- my.ownsite@ourearth.org
- mysite@you.me.net
- santosh@gmail.com
- biveka@gmail.com
Example of invalid email id
- mysite.ourearth.com [@ is not present]
- mysite@.com.my [ tld (Top Level domain) can not start with dot "." ]
- @you.me.net [ No character before @ ]
- mysite123@gmail.b [ ".b" is not a valid tld ]
- mysite@.org.org [ tld can not start with dot "." ]
- .mysite@mysite.org [ an email should not be start with "." ]
- mysite()*@gmail.com [ here the regular expression only allows character, digit, underscore, and dash ]
- mysite..1234@yahoo.com [double dots are not allowed]
JavaScript code to validate an email id
To get a valid email id we use a regular expression /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/. According to http://tools.ietf.org/html/rfc3696#page-5 ! # $ % & ‘ * + – / = ? ^ ` . { | } ~ characters are legal in the local part of an e-mail address but in the above regular expression those characters are filtered out. You can modify or rewrite the said regular expression.
Flowchart :
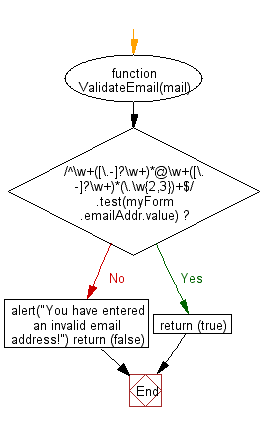
Explanation of the said Regular expression (email id)
Regular Expression Pattern
/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/
Character | Description |
---|---|
/ .. / | All regular expressions start and end with forward slashes. |
^ | Matches the beginning of the string or line. |
\w+ | Matches one or more word characters including the underscore. Equivalent to [A-Za-z0-9_]. |
[\.-] | \ Indicates that the next character is special and not to be interpreted literally. .- matches character . or -. |
? | Matches the previous character 0 or 1 time. Here previous character is [.-]. |
\w+ | Matches 1 or more word characters including the underscore. Equivalent to [A-Za-z0-9_]. |
* | Matches the previous character 0 or more times. |
([.-]?\w+)* | Matches 0 or more occurrences of [.-]?\w+. |
\w+([.-]?\w+)* | The sub-expression \w+([.-]?\w+)* is used to match the username in the email. It begins with at least one or more word characters including the underscore, equivalent to [A-Za-z0-9_]. , followed by . or - and . or - must follow by a word character (A-Za-z0-9_). |
@ | It matches only @ character. |
\w+([.-]?\w+)* | It matches the domain name with the same pattern of user name described above.. |
\.\w{2,3} | It matches a . followed by two or three word characters, e.g., .edu, .org, .com, .uk, .us, .co etc. |
+ | The + sign specifies that the above sub-expression shall occur one or more times, e.g., .com, .co.us, .edu.uk etc. |
$ | Matches the end of the string or line. |
Note: If you want to work on 4 digit domain, for example, .info then you must change w{2,3} to w{2,4}.
Let apply the above JavaScript function in an HTML form.
HTML Code
JavaScript Code
CSS Code
No comments:
Post a Comment